Python Tutorials
Python has a variety of built-in exceptions for handling exceptions, but it is occasionally necessary to define unique exceptions that are suited to the particular requirements of a project. We will examine user-defined exceptions in Python, their definition, and the significance of comprehending them in this article.
As the name implies, user-defined exceptions are exceptions made by a user to address particular scenarios in their code. By defining a new class that derives from the built-in Exception class or one of its subclasses, programmers can create unique exceptions in Python.
For writing reliable and error-free code, it is essential to comprehend how to define and use user-defined exceptions. Custom exceptions give programmers the ability to deliver thorough error messages that can aid in the runtime diagnosis of problems and facilitate their resolution. Additionally, because they offer a way to arrange and encapsulate error handling logic, custom exceptions can improve the readability and maintainability of code.
We will first go over what user-defined exceptions are, why they are helpful, and a few Python usage examples in this article. The process of developing unique exception classes will then be discussed, including issues like deriving from built-in exceptions and overriding parent class methods. To help you understand how to use custom exception classes in your own code, we’ll give you a few examples of how to create them.
Looking to Learn Python? Book a Free Trial Lesson and match with top Python Tutors for concepts, projects and assignment help on Wiingy today!
What are User-Defined Exceptions in Python?
A. Definition of user-defined exceptions
User-defined exceptions are ones that the developer writes in their code to address particular errors. These exceptions are defined by creating a new class that inherits from the Exception class or one of its subclasses.
B. Why use user-defined exceptions?
There are several reasons why developers might want to use user-defined exceptions in their code. One of the main reasons is that they allow for more detailed and specific error messages to be provided to the user. This can help with debugging and makes it easier to fix errors in the code. Additionally, user-defined exceptions can help with code organization and encapsulation, making it easier to maintain and update the codebase over time.
C. Examples of user-defined exceptions in Python
Example of creating a custom exception class
class InvalidInputError(Exception):
pass
In this example, we define a custom exception class called InvalidInputError that inherits from the built-in Exception class. The pass keyword is used to indicate that the class doesn’t contain any additional logic or properties beyond what is provided by its parent class.
Example of raising a user-defined exception
def divide_numbers(a, b):
if b == 0:
raise ZeroDivisionError("Cannot divide by zero")
if not isinstance(a, int) or not isinstance(b, int):
raise TypeError("Inputs must be integers")
return a / b
In this example, we define a function divide_numbers that takes two arguments, a and b, and returns the result of dividing a by b. If the value of b is 0 or if a or b are not integers, we raise custom exceptions ZeroDivisionError and TypeError, respectively. These exceptions provide more specific error messages than the built-in exceptions would provide.
Creating Custom Exception Classes
A. Defining a custom exception class
To define a custom exception class in Python, we begin by creating a new class that inherits from Exception or one of its subclasses. The class can then receive additional logic and properties as required.
For example, let’s say we want to create a custom exception class to handle invalid email addresses:
class InvalidEmailError(Exception):
def __init__(self, email):
self.email = email
def __str__(self):
return f"{self.email} is not a valid email address"
In this example, we define a custom exception class called InvalidEmailError that inherits from the Exception class. We also define two methods for the class – __init__ and __str__.
The __init__ method is used to initialize the email property of the exception instance. This method takes an email address as a parameter and assigns it to the email property.
The __str__ method is used to define a string representation of the exception instance. In this case, we return a string that includes the invalid email address that caused the exception.
B. Inheriting from built-in exceptions
It’s typical to inherit from one of Python’s built-in exception classes when developing a custom exception class. This may offer extra features and qualities that are helpful for handling errors.
For example, let’s say we want to create a custom exception class to handle invalid URLs. We can inherit from the built-in ValueError class, which is commonly used for input validation errors:
class InvalidURLError(ValueError):
def __init__(self, url):
self.url = url
def __str__(self):
return f"{self.url} is not a valid URL"
In this example, we define a custom exception class called InvalidURLError that inherits from the ValueError class. We also define two methods for the class – __init__ and __str__ – similar to the previous example.
C. Overriding methods of the parent class
When inheriting from a built-in exception class, it’s common to override some of the methods defined by the parent class. This allows us to customize the behavior of the exception to better suit our needs.
For example, let’s say we want to create a custom exception class to handle errors related to file operations. We can inherit from the built-in IOError class and override the __str__ method to provide a more detailed error message:
class FileError(IOError):
def __init__(self, filename):
self.filename = filename
def __str__(self):
return f"Error accessing file: {self.filename}"
In this example, we define a custom exception class called FileError that inherits from the IOError class. We override the __str__ method to return a string that includes the filename of the file that caused the exception.
D. Examples of creating custom exception classes
Here are a few more examples of creating custom exception classes in Python:
class InvalidAgeError(Exception):
def __init__(self, age):
self.age = age
def __str__(self):
return f"{self.age} is not a valid age"
class InsufficientFundsError(ValueError):
def __init__(self, balance, amount):
self.balance = balance
self.amount = amount
def __str__(self):
return f"Insufficient funds: balance={self.balance}, amount={self.amount}"
class InvalidDateFormatError(ValueError):
def __init__(self, date_str):
self.date_str = date_str
def __str__(self):
return f"{self.date_str} is not a valid date format"
In these examples, we define custom exception classes to handle errors related to
Raising User-Defined Exceptions
A. When to raise user-defined exceptions
User-defined exceptions should be raised when a specific error condition occurs in your code that is not covered by the built-in exceptions. This can include situations such as invalid input values, unexpected behavior, or any other scenario that is specific to your code.
B. How to raise user-defined exceptions
To raise a user-defined exception in Python, you simply need to create an instance of the exception class and then use the raise keyword to raise the exception. You can optionally pass additional data or a custom error message to the exception instance.
C. Examples of raising user-defined exceptions
Example of raising a custom exception with a message
def calculate_discount(price, discount_rate):
if discount_rate < 0 or discount_rate > 1:
raise ValueError("Discount rate must be between 0 and 1")
return price * (1 - discount_rate)
In this example, we define a function calculate_discount that takes two arguments, price and discount_rate, and calculates the discounted price. If the discount rate is not between 0 and 1, we raise a ValueError exception with a custom error message.
Example of raising a custom exception with additional data
class InvalidInputError(Exception):
def __init__(self, message, input_value):
self.message = message
self.input_value = input_value
def __str__(self):
return f"{self.message}: {self.input_value}"
def divide_numbers(a, b):
if b == 0:
raise ZeroDivisionError("Cannot divide by zero")
if not isinstance(a, int) or not isinstance(b, int):
raise InvalidInputError("Inputs must be integers", (a, b))
return a / b
In this example, we define a custom exception class InvalidInputError that takes a message and an input value as arguments. We then define a function divide_numbers that takes two arguments, a and b, and returns the result of dividing a by b. If either a or b is not an integer, we raise an InvalidInputError exception with the inputs as additional data.
Catching User-Defined Exceptions
A. Handling user-defined exceptions
The try and except keywords in Python can be used to handle a user-defined exception. You place the code that might cause an exception in the try block, and you place the code that should run if the exception is raised in the except block.
B. Multiple except blocks for different exceptions
To handle various exception types, you can use multiple except blocks. As a result, you can give each type of exception its own unique error handling logic.
C. Catching the base exception class
To handle any exception that might be raised, you can also catch the base Exception class. Instead of catching the base exception class, it is typically advised to catch specific exceptions because doing so can make it more difficult to debug programming errors.
D. Examples of catching user-defined exceptions
try:
result = divide_numbers(10, "5")
except InvalidInputError as e:
print(f"Invalid input: {e}")
except ZeroDivisionError as e:
print(f"Cannot divide by zero: {e}")
except Exception as e:
print(f"An error occurred: {e}")
In this example, we use a try block to call the divide_numbers function with the arguments 10 and “5”. If the inputs are invalid, the `Invalid
Conclusion
We have covered the Python idea of user-defined exceptions in this article. User-defined exceptions are exceptions created by the developer to handle specific scenarios in their code. We have explained why they are important and provided examples of how to create and raise custom exception classes. Additionally, we have discussed how to handle user-defined exceptions using try and except blocks, and provided some best practices to follow when using user-defined exceptions in your code.
User-defined exceptions should be used properly to increase your code’s readability and maintainability. They enable the user to receive more granular and targeted error messages, which can aid in error debugging and repair. Additionally, they allow for error handling logic to be encapsulated and organized, making it easier to maintain and update the codebase over time.
Overall, understanding how to create and use user-defined exceptions is an important skill for any Python developer. By following best practices and using user-defined exceptions appropriately, you can greatly improve the quality and reliability of your code.
Looking to Learn Python? Book a Free Trial Lesson and match with top Python Tutors for concepts, projects and assignment help on Wiingy today!
FAQs
What is user-defined exception in Python?
In Python, a user-defined exception is one that the developer has written to address particular use cases.
What is a user-defined exception?
An exception that has been written by the developer to address particular scenarios in their code is known as a user-defined exception.
What are the 4 types of exceptions in Python?
In Python, there are four different exception types: SyntaxError, TypeError, NameError, and ValueError.
What is user-defined exception in Python Tutorialspoint?
User-defined exceptions in Python are exceptions created by the developer to handle specific scenarios in their code. They are covered in-depth in the Python Tutorialspoint documentation
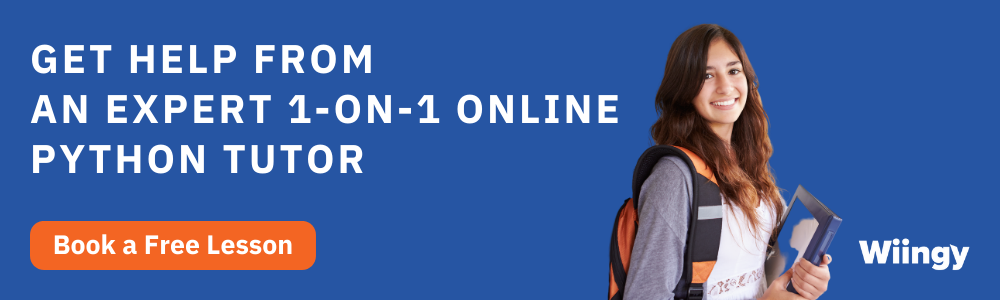
Written by
Rahul LathReviewed by
Arpit Rankwar